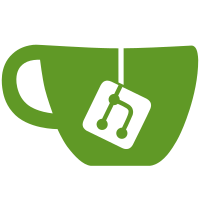
-----BEGIN PGP SIGNATURE----- iQJEBAABCgAuFiEEA5IzWngIOJSkMBxDI26KWMbbRRIFAmENYHwQHG1heEBtdXNp Y3BkLm9yZwAKCRAjbopYxttFEpGtD/9ToU27x36NAAFpChicSqbu3h2wtJ29lowT ivV80XB26pQeGK0DSXADSs38MVXo42i3vqg0zGWV9TRbcDs5VErXANVLN16qsKCu U0v1BDY11UiYp6ATiUjIahyG1UsmbRVZlfDyVIhYvmFpGLFw6+03HH6w2k/v85ns FxyXkYDYcUokPJPEQcChE1eIwKsGM6MjbdVIkJAEo3wGhL/Hhy2KUskrFcCo1PDj 7xxGrwauG+8wSjAWMA5vzl3udRaDauuXztm5QbQIDdsbRaCiBAdgkzC0PvIxTOr0 bR4WHVB0KSiM96yIXNtg/WZxO0XrxppmX/E4eZSgz0JGKMrAHcoTJAUCIDu3X719 gJnJLg7r2X5dTchXezv09YoJolKbw1bOooyAuE4FCDWMsOa2GRuBZC+8w7DNTZuo PTh+Z40fnfpNBofe+e/WZrXr6i2TKk8CqHEidq2GHlOkvTR7g6m8MjOLvZNHotMb 9ECr9MhzXH+nvEX8IaxvjWsfLJiDbUgnVsQ+6akGtkbragaDN/Wgr/XdkELoLlsK LZFY5ngnZmDXOu4tjBLJtfrTkZB2/Hld4xtF1qlsy9fvZBRKeKlpABTLaa8r/vnR Ta0rB2O3/dculZbHZRUnZvLS4Xv4g322vW9Wso9IBoWRn9fC8b2zR93k7breAqcn pKbYGg/j6g== =/954 -----END PGP SIGNATURE----- Merge tag 'v0.22.10' release v0.22.10
119 lines
3.4 KiB
C++
119 lines
3.4 KiB
C++
/*
|
|
* Copyright 2003-2021 The Music Player Daemon Project
|
|
* http://www.musicpd.org
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
*/
|
|
|
|
#include "Walk.hxx"
|
|
#include "UpdateDomain.hxx"
|
|
#include "db/DatabaseLock.hxx"
|
|
#include "db/PlaylistVector.hxx"
|
|
#include "db/plugins/simple/Directory.hxx"
|
|
#include "lib/fmt/ExceptionFormatter.hxx"
|
|
#include "song/DetachedSong.hxx"
|
|
#include "input/InputStream.hxx"
|
|
#include "playlist/PlaylistPlugin.hxx"
|
|
#include "playlist/PlaylistRegistry.hxx"
|
|
#include "playlist/PlaylistStream.hxx"
|
|
#include "playlist/SongEnumerator.hxx"
|
|
#include "storage/FileInfo.hxx"
|
|
#include "storage/StorageInterface.hxx"
|
|
#include "fs/Traits.hxx"
|
|
#include "util/StringFormat.hxx"
|
|
#include "Log.hxx"
|
|
|
|
void
|
|
UpdateWalk::UpdatePlaylistFile(Directory &parent, std::string_view name,
|
|
const StorageFileInfo &info,
|
|
const PlaylistPlugin &plugin) noexcept
|
|
{
|
|
assert(plugin.open_stream);
|
|
|
|
Directory *directory =
|
|
LockMakeVirtualDirectoryIfModified(parent, name, info,
|
|
DEVICE_PLAYLIST);
|
|
if (directory == nullptr)
|
|
/* not modified */
|
|
return;
|
|
|
|
const auto uri_utf8 = storage.MapUTF8(directory->GetPath());
|
|
|
|
FmtDebug(update_domain, "scanning playlist '{}'", uri_utf8);
|
|
|
|
try {
|
|
Mutex mutex;
|
|
auto e = plugin.open_stream(InputStream::OpenReady(uri_utf8.c_str(),
|
|
mutex));
|
|
if (!e) {
|
|
/* unsupported URI? roll back.. */
|
|
editor.LockDeleteDirectory(directory);
|
|
return;
|
|
}
|
|
|
|
unsigned track = 0;
|
|
|
|
while (true) {
|
|
auto song = e->NextSong();
|
|
if (!song)
|
|
break;
|
|
|
|
auto db_song = std::make_unique<Song>(std::move(*song),
|
|
*directory);
|
|
db_song->target =
|
|
PathTraitsUTF8::IsAbsoluteOrHasScheme(db_song->filename.c_str())
|
|
? db_song->filename
|
|
/* prepend "../" to relative paths to
|
|
go from the virtual directory
|
|
(DEVICE_PLAYLIST) to the containing
|
|
directory */
|
|
: "../" + db_song->filename;
|
|
db_song->filename = StringFormat<64>("track%04u",
|
|
++track);
|
|
|
|
{
|
|
const ScopeDatabaseLock protect;
|
|
directory->AddSong(std::move(db_song));
|
|
}
|
|
}
|
|
} catch (...) {
|
|
FmtError(update_domain,
|
|
"Failed to scan playlist '{}': {}",
|
|
uri_utf8, std::current_exception());
|
|
editor.LockDeleteDirectory(directory);
|
|
}
|
|
}
|
|
|
|
bool
|
|
UpdateWalk::UpdatePlaylistFile(Directory &directory,
|
|
std::string_view name, std::string_view suffix,
|
|
const StorageFileInfo &info) noexcept
|
|
{
|
|
const auto *const plugin = FindPlaylistPluginBySuffix(suffix);
|
|
if (plugin == nullptr)
|
|
return false;
|
|
|
|
if (GetPlaylistPluginAsFolder(*plugin))
|
|
UpdatePlaylistFile(directory, name, info, *plugin);
|
|
|
|
PlaylistInfo pi(name, info.mtime);
|
|
|
|
const ScopeDatabaseLock protect;
|
|
if (directory.playlists.UpdateOrInsert(std::move(pi)))
|
|
modified = true;
|
|
|
|
return true;
|
|
}
|